Curriculum
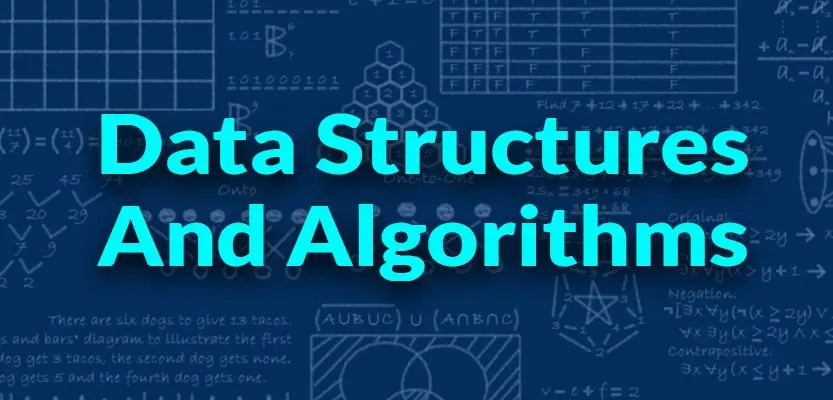
Eureka Data Academy Bootcamp equips professionals with highly applicable skills in data structures and algorithms, essential for solving complex problems across any industry.
It’s simple: Companies value what you can accomplish, not just what you claim to know. That’s why our curriculum focuses on applying your knowledge to real-world challenges, such as optimizing search algorithms or designing efficient systems for large-scale data processing. Our students master the art of building, navigating, and optimizing data structures and algorithms to create efficient, scalable solutions that employers demand.
This 12-week curriculum caters to students, working professionals, and individuals preparing for technical interviews. Whether you are new to Data Structures and Algorithms (DSA) or looking to refine your Python-based problem-solving skills, this course provides a structured path to mastering key concepts. With a balance of theory and hands-on activities, you’ll build a strong foundation and confidently tackle real-world problems and coding interviews.
Students looking to scale through technical interviews will have a bonus week after the 12 weeks of training. This bonus week will support students specifically taking this course with the end goal of cracking the coding interview.
Introduction To Dsa And Python FundamentalsWeek 1
Description:
What You Will Learn:
Topics:
- Introduction to Data Structures and Algorithm
- Definitions and significance.
- Real-world applications of DSA.
- Python Basics
- Data types: integers, strings, lists, dictionaries, sets, and tuples.
- Control structures: if-else, loops (for, while).
- Functions and scope.
- Time Complexity
- Big-O notation.
- Analyze time complexity of simple loops.
Activities
- Write a Python function to calculate factorial using iteration.
- Implement a function to calculate the sum of elements in a list and analyze its time complexity.
Arrays, Strings, Dictionaries And SetsWeek 2
Description:
What You Will Learn:
Topics:
- Arrays
- Basics, indexing, slicing.
- Common operations: insert, delete, search.
- Strings
- String manipulation and slicing.
- Built-in functions like find, join, replace.
- Problems
- Reverse an array or string.
- Check if a string is a palindrome.
Exercises
- Reverse Words in a String
- Valid Word
- Rotate Array
- Two Sum
- Group Anagrams
- Ransom Note
- Intersection of Two Arrays
Stacks And QueuesWeek 3
Description:
What You Will Learn:
Topics:
- Stacks
- LIFO principle.
- Use cases: undo operations, function calls.
- Queues
- FIFO principle.
- Circular queue implementation.
- Problems
- Valid Parentheses (using stack).
- Implement "Next Greater Element" using stack.
Exercises
- Evaluate Reverse Polish Notation
- Next Greater Element I
Linked ListsWeek 4
Description:
What You Will Learn:
Topics
- Singly Linked Lists
- Create, traverse, insert, delete nodes.
- Applications and problems
- Detect a loop in a linked list.
- Reverse a linked list.
Exercises
- Merge Two Sorted Lists
- Delete Node in a Linked List
- Middle of the Linked List
Recursion And BacktrackingWeek 5
Description:
What You Will Learn:
Topics
- Recursion
- Base cases, recursive calls.
- Examples: factorial, Fibonacci.
- Backtracking
- Solving constraint-based problems.
- Problems
- N-Queens problem.
- Generate all subsets of a set.
Exercises
- Fibonacci Number
- Generate Parentheses
Binary TreesWeek 6
Description:
What You Will Learn:
Topics
- Binary Trees
- Structure and node representation.
- Traversals: inorder, preorder, postorder.
- Binary Tree problems:
- Height of a tree.
- Level order traversal.
Exercises
- Binary Tree Inorder Traversal
- Binary Tree Preorder Traversal
- Binary Tree Postorder Traversal
- Invert Binary Tree
Binary Search Trees (Bsts)Week 7
Description:
What You Will Learn:
Topics
- Properties of BSTs.
- Operations
- Insert, search, delete.
- Applications
- Validate a BST.
- Find the Kth smallest element.
Exercises
- Convert Sorted Array to BST
- Search in a Binary Search Tree
Heaps And Priority QueuesWeek 8
Description:
What You Will Learn:
Topics
- Min-Heap and Max-Heap.
- Python’s heapq module for heap operations.
- Problems
- Heap Sort.
- Merge K Sorted Lists.
Exercises
- Kth Largest Element in an Array
- Top K Frequent Elements
Graphs – BasicsWeek 9
Description:
What You Will Learn:
Topics
- Graph Representation
- Adjacency matrix and adjacency list.
- Traversal Algorithms
- Depth First Search (DFS).
- Breadth First Search (BFS).
- Problems
- Detect cycles in a graph.
- Find connected components.
Exercises
- Find if Path Exists in Graph
- Find the Town Judge
Graphs – AdvancedWeek 10
Description:
What You Will Learn:
Topics
- Advanced Graph Algorithms
- Dijkstra’s algorithm for shortest paths.
- Topological sort for Directed Acyclic Graphs (DAGs).
- Problems
- Minimum Spanning Tree (using Kruskal’s or Prim’s algorithm).
- Course Scheduling (topological sorting).
Exercises
- Shortest Path in Binary Matrix
- Number of Provinces
Searching And SortingWeek 11
Description:
What You Will Learn:
Topics
- Binary Search
- Applications and edge cases.
- Sorting Algorithms
- Quick Sort and Merge Sort.
- Problems
- Search in Rotated Sorted Array.
- Find Peak Element.
Exercises
- Squares of a Sorted Array
- Kth Largest Element in an Array
- Merge Sorted Array
- Missing Number
- Two Sum II - Input Array Is Sorted
Dynamic ProgrammingWeek 12
Description:
What You Will Learn:
Topics
- Introduction to Dynamic Programming (DP)
- Memoization and tabulation.
- DP Problems
- Longest Common Subsequence.
- Knapsack problem.
- Common DP Patterns
- Subset Sum.
- Climbing Stairs.
Exercises
- Coin Change
- Longest Increasing Subsequence
- Maximum Subarray
- Best Time to Buy and Sell Stock
- Coin Change II
Bonus Week (Optional)Week 13
Description:
What You Will Learn:
Technical Interview Prep Discussion and Hands-on